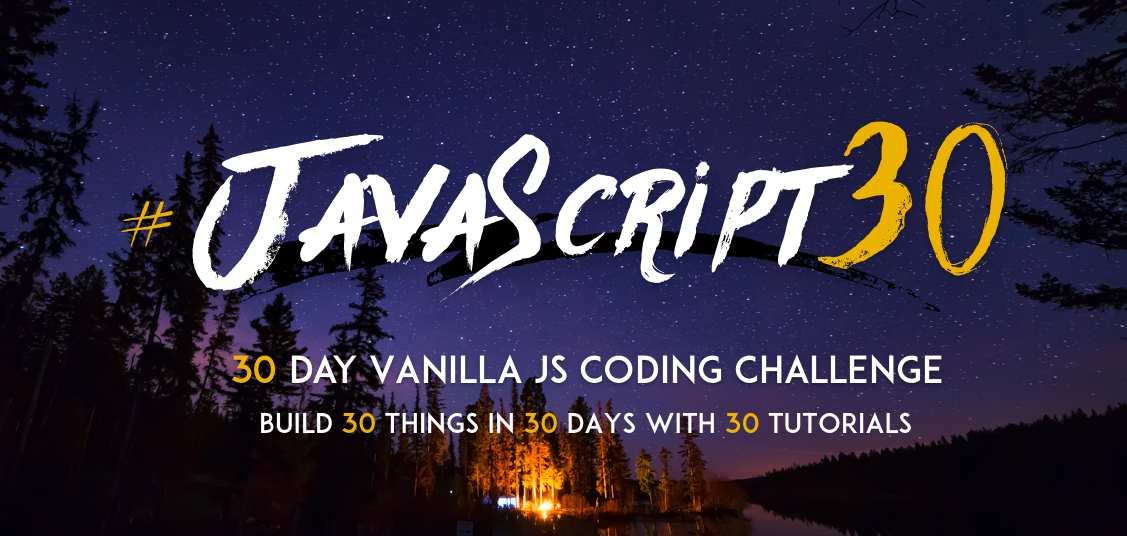
안녕하세요 오늘도 자스 연습을 해보도록 하겠습니다
JavaScript 30
Build 30 things with vanilla JS in 30 days with 30 tutorials
javascript30.com
목차 바로가기
1. 오늘의 과제는? |
2. 내가 만든 파일 |
3. finished파일 리뷰 |
4. 배운 점 & 느낀 점 |
1. 오늘의 과제는?
오늘은 몇가지 배열 같은 데이터들을 이용해서 자바스크립트 배열과 관련된 함수를 써보는 과제입니다.
start 파일의 주석을 읽어보시면 데이터를 가지고 뭘 해야하는지, 어떤 함수를 써야하는지까지 나와있습니다. 이를 보시고 finished 파일처럼 작동하게 만드시면 됩니다.
start.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Array Cardio 💪</title>
</head>
<body>
<p><em>Psst: have a look at the JavaScript Console</em> 💁</p>
<script>
// Get your shorts on - this is an array workout!
// ## Array Cardio Day 1
// Some data we can work with
const inventors = [
{ first: 'Albert', last: 'Einstein', year: 1879, passed: 1955 },
{ first: 'Isaac', last: 'Newton', year: 1643, passed: 1727 },
{ first: 'Galileo', last: 'Galilei', year: 1564, passed: 1642 },
{ first: 'Marie', last: 'Curie', year: 1867, passed: 1934 },
{ first: 'Johannes', last: 'Kepler', year: 1571, passed: 1630 },
{ first: 'Nicolaus', last: 'Copernicus', year: 1473, passed: 1543 },
{ first: 'Max', last: 'Planck', year: 1858, passed: 1947 },
{ first: 'Katherine', last: 'Blodgett', year: 1898, passed: 1979 },
{ first: 'Ada', last: 'Lovelace', year: 1815, passed: 1852 },
{ first: 'Sarah E.', last: 'Goode', year: 1855, passed: 1905 },
{ first: 'Lise', last: 'Meitner', year: 1878, passed: 1968 },
{ first: 'Hanna', last: 'Hammarström', year: 1829, passed: 1909 }
];
const people = ['Beck, Glenn', 'Becker, Carl', 'Beckett, Samuel', 'Beddoes, Mick', 'Beecher, Henry', 'Beethoven, Ludwig', 'Begin, Menachem', 'Belloc, Hilaire', 'Bellow, Saul', 'Benchley, Robert', 'Benenson, Peter', 'Ben-Gurion, David', 'Benjamin, Walter', 'Benn, Tony', 'Bennington, Chester', 'Benson, Leana', 'Bent, Silas', 'Bentsen, Lloyd', 'Berger, Ric', 'Bergman, Ingmar', 'Berio, Luciano', 'Berle, Milton', 'Berlin, Irving', 'Berne, Eric', 'Bernhard, Sandra', 'Berra, Yogi', 'Berry, Halle', 'Berry, Wendell', 'Bethea, Erin', 'Bevan, Aneurin', 'Bevel, Ken', 'Biden, Joseph', 'Bierce, Ambrose', 'Biko, Steve', 'Billings, Josh', 'Biondo, Frank', 'Birrell, Augustine', 'Black, Elk', 'Blair, Robert', 'Blair, Tony', 'Blake, William'];
// Array.prototype.filter()
// 1. Filter the list of inventors for those who were born in the 1500's
// Array.prototype.map()
// 2. Give us an array of the inventors' first and last names
// Array.prototype.sort()
// 3. Sort the inventors by birthdate, oldest to youngest
// Array.prototype.reduce()
// 4. How many years did all the inventors live?
// 5. Sort the inventors by years lived
// 6. create a list of Boulevards in Paris that contain 'de' anywhere in the name
// https://en.wikipedia.org/wiki/Category:Boulevards_in_Paris
// 7. sort Exercise
// Sort the people alphabetically by last name
// 8. Reduce Exercise
// Sum up the instances of each of these
const data = ['car', 'car', 'truck', 'truck', 'bike', 'walk', 'car', 'van', 'bike', 'walk', 'car', 'van', 'car', 'truck' ];
</script>
</body>
</html>
보시는바와 같이 오늘은 html과 css가 중요하지 않습니다. Only JS에만 집중하시면 될거같습니다.
2. 내가 만든 파일
// Array.prototype.filter()
// 1. Filter the list of inventors for those who were born in the 1500's
const inventors_1500 = inventors.filter(inventor => {
if(inventor.year >= 1500 && inventor.year < 1600) return inventor;
});
console.log(inventors_1500);
우선 첫번째로 1500년대에 태어난 발명가들의 리스트를 필터링하는 것입니다. 화살표함수를 통해서 1500~1599년에 태어난 사람을 선별해서 출력했습니다. 필터 메서드를 통해서 리스트를 반환하는 것을 확인할 수 있었습니다.
// Array.prototype.map()
// 2. Give us an array of the inventors' first and last names
const inventorsName = inventors.map(inventor => {
return [inventor.first, inventor.last];
});
console.log(inventorsName);
발명가의 첫번째 마지막 이름의 배열을 출력하는 것입니다. map() 메서드를 통해서 배열의 모든 요소에 접근할 수 있었고, 각 요소의 first와 last를 가져와서 배열로 반환했습니다. 나중에 완성본 파일 보니까 그냥 중첩배열말고 문자열의 배열로 해도 괜찮았나 봅니다.
// Array.prototype.sort()
// 3. Sort the inventors by birthdate, oldest to youngest
const sortedInventors = inventors.sort(function (a, b) {
if(a.year > b.year) {
return -1;
}
else if(a.year < b.year) {
return 1;
}
else {
return 0;
}
});
console.log(sortedInventors);
태어난 년도를 활용해서 오름차순이나 내림차순으로 정렬하면 됩니다. 저는 내림차순으로 정렬하기 위해서 a.year가 더 클 때 -1 을 반환해서 a가 먼저 오게 만들었습니다.
// Array.prototype.reduce()
// 4. How many years did all the inventors live?
const inventorsLivedYears = inventors.reduce(function (total, inventor) {
return total + (inventor.passed - inventor.year);
}, 0);
console.log(inventorsLivedYears);
다음으로 reduce() 메서드입니다. 발명가들이 살았던 기간들의 총합을 구하면 됩니다.
reduce는 좀 이해가 안갔었는데 우리가 1부터 10까지의 합을 구할 때 변수 sum같은 것을 이용해서 값들을 쌓는식으로 총합을 구하는데 그런것과 비슷한 느낌인거같습니다.
그래서 total에 inventor가 살았던 기간(inventor.passed - inventor.year)를 모두 더합니다. 그리고 total의 초기값으로 0으로 준것을 확인할 수 있습니다.
// 5. Sort the inventors by years lived
const sortedByLivedYears = inventors.sort(function (a, b) {
aLived = a.passed - a.year;
bLived = b.passed - b.year;
if(aLived > bLived) {
return -1;
}
else if(aLived < bLived) {
return 1;
}
else {
return 0;
}
});
console.log(sortedByLivedYears);
살았던 기간을 기준으로 sort를 하면 됩니다. 저는 내림차순으로 했습니다.
6번째는 어떻게 하는지 몰라서 못했습니다.
// 7. sort Exercise
// Sort the people alphabetically by last name
const sortedPeople = people.sort((previOne, nextOne) => {
const a = previOne.split(", ");
const b = nextOne.split(", ");
if(a[0] > b[0]) {
return 1;
}
else if(a[0] < b[0]) {
return -1;
}
else {
return 0;
}
});
console.log(sortedPeople);
Last name을 기준으로 알파벳순으로 정렬하면 됩니다. 우선 people배열이
const people = ['Beck, Glenn', 'Becker, Carl', 'Beckett, Samuel', 'Beddoes, Mick', 'Beecher, Henry', 'Beethoven, Ludwig', 'Begin, Menachem', 'Belloc, Hilaire', 'Bellow, Saul', 'Benchley, Robert', 'Benenson, Peter', 'Ben-Gurion, David', 'Benjamin, Walter', 'Benn, Tony', 'Bennington, Chester', 'Benson, Leana', 'Bent, Silas', 'Bentsen, Lloyd', 'Berger, Ric', 'Bergman, Ingmar', 'Berio, Luciano', 'Berle, Milton', 'Berlin, Irving', 'Berne, Eric', 'Bernhard, Sandra', 'Berra, Yogi', 'Berry, Halle', 'Berry, Wendell', 'Bethea, Erin', 'Bevan, Aneurin', 'Bevel, Ken', 'Biden, Joseph', 'Bierce, Ambrose', 'Biko, Steve', 'Billings, Josh', 'Biondo, Frank', 'Birrell, Augustine', 'Black, Elk', 'Blair, Robert', 'Blair, Tony', 'Blake, William'];
이렇게 생겼습니다. 따라서 저기 보이는 맨 처음 이름인 "Beck, Glenn"을 예를 들자면 split(", ")을 통해서 Beck과 Glenn으로 나눠서 Beck을 선택해서 이것을 기준으로 정렬해야합니다.
따라서 split(", ")을 하게되면 ["Beck", "Glenn"] 이라는 배열이 반환될 것이고 따라서 배열의 인덱스 0번째를 선택해야합니다.
제가 쓴 코드에서도 잘 반영되어 있습니다. a와 b에서 각각 두개의 단어배열을 받아서 0번째 요소로 알파벳순서를 비교합니다. 그렇게 해서 사전상으로 작은게 먼저 오도록 정렬했습니다.
// 8. Reduce Exercise
// Sum up the instances of each of these
const data = ['car', 'car', 'truck', 'truck', 'bike', 'walk', 'car', 'van', 'bike', 'walk', 'car', 'van', 'car', 'truck' ];
const sumUpData = data.reduce(function(obj, item) {
if (!obj[item]) {
obj[item] = 0;
}
obj[item]++;
return obj;
}, {});
console.log(sumUpData);
data 배열을 요약하는 과제인데 어떻게 하는지 몰라서 finished 버전을 보고 이해하였습니다. 3번째 단락 finished파일 리뷰에서 설명을 해보겠습니다.
3. finished파일 리뷰
// Array.prototype.filter()
// 1. Filter the list of inventors for those who were born in the 1500's
const fifteen = inventors.filter(inventor => (inventor.year >= 1500 && inventor.year < 1600));
console.table(fifteen);
화살표 함수를 사용해서 더 간단하게 결과를 출력한 것을 볼 수 있습니다.
// Array.prototype.map()
// 2. Give us an array of the inventor first and last names
const fullNames = inventors.map(inventor => `${inventor.first} ${inventor.last}`);
console.log(fullNames);
map을 통해서 배열의 요소들을 하나하나 돌면서 리터럴 문자열을 이용해서 간단하게 결과를 출력했습니다.
// Array.prototype.sort()
// 3. Sort the inventors by birthdate, oldest to youngest
// const ordered = inventors.sort(function(a, b) {
// if(a.year > b.year) {
// return 1;
// } else {
// return -1;
// }
// });
const ordered1 = inventors.sort((a, b) => a.year > b.year ? 1 : -1);
console.table(ordered1);
삼항연산자를 통해서 더 a.year가 더 크면 1을 반환해서 b가 먼저 나올 수 있게 sort() 메서드를 쓴 것을 볼 수 있습니다. 그리고 console.table() 를 쓴 것을 볼 수 있습니다.
// Array.prototype.reduce()
// 4. How many years did all the inventors live?
const totalYears = inventors.reduce((total, inventor) => {
return total + (inventor.passed - inventor.year);
}, 0);
console.log(totalYears);
설명 생략
// 5. Sort the inventors by years lived
const oldest = inventors.sort(function(a, b) {
const lastInventor = a.passed - a.year;
const nextInventor = b.passed - b.year;
return lastInventor > nextInventor ? -1 : 1;
});
console.table(oldest);
제가 한 것에서 삼항 연산자를 통해서 결과를 얻어낸 모습입니다.
삼항연산자가 확실히 더 깔끔해 보이는 것을 볼 수 있습니다. 저도 삼항연산자를 자주 써봐야겠네요
// 6. create a list of Boulevards in Paris that contain 'de' anywhere in the name
// https://en.wikipedia.org/wiki/Category:Boulevards_in_Paris
// const category = document.querySelector('.mw-category');
// const links = Array.from(category.querySelectorAll('a'));
// const de = links
// .map(link => link.textContent)
// .filter(streetName => streetName.includes('de'));
저 사이트에 들어가서 주석처리된 코드를 입력하시면 이름에 'de' 가 들어간 Boulevards in Paris의 배열을 얻을 수 있습니다.
.mv-category 클래스를 써서 각 a태그들이 담겨있는 박스를 가져와서 category상수에 저장합니다. 그리고 그 category에서 a태그를 가진 객체들을 모두 가져와서 배열로 만든 것을 links에 저장합니다.
links에서 map을 통해서 모든 배열요소들을 돌면서 a태그 객체에 담겨있는 textContent들의 배열을 만듭니다.
그리고 filter 메서드를 통해서 각 요소들에서 'de'가 들어간 요소들의 배열을 반환합니다.
직접 해당 사이트에서 크롬개발도구(F12)를 써서 해보시면 이해가 더 쉬울것입니다.
// 7. sort Exercise
// Sort the people alphabetically by last name
const alpha = people.sort((lastOne, nextOne) => {
const [aLast, aFirst] = lastOne.split(', ');
const [bLast, bFirst] = nextOne.split(', ');
return aLast > bLast ? 1 : -1;
});
console.log(alpha);
설명 생략
// 8. Reduce Exercise
// Sum up the instances of each of these
const data = ['car', 'car', 'truck', 'truck', 'bike', 'walk', 'car', 'van', 'bike', 'walk', 'car', 'van', 'car', 'truck' ];
const sumUpData = data.reduce(function(obj, item) {
if (!obj[item]) {
obj[item] = 0;
}
obj[item]++;
return obj;
}, {});
console.log(sumUpData);
data 배열을 요약하는 객체를 출력하면 됩니다.
우선 function(obj, item) 으로 진행했는데 여기서 obj의 초기값으로 {}를 줬습니다. 따라서 객체가 될 수 있었습니다.
그리고 item에는 각 배열의 요소들이 담기게 될 것인데 예를 들어 맨 처음엔 obj['car']가 될 수 있습니다.
하지만 객체에 없었던 것이므로 if 조건에 맞아떨어집니다. 따라서 obj[item] = 0; 을 통해서 객체의 요소로 설정되고 obj[item]++; 로 개수가 설정됩니다.
그리고 reduce() 메서드 이므로 계속 중첩되고 결과적으로 요약이 출력됩니다.
4. 배운 점 & 느낀 점
em태그 - 강조된 텍스트를 표현할 때 사용 - http://tcpschool.com/html-tags/em |
Array.prototype.filter() -https://developer.mozilla.org/ko/docs/Web/JavaScript/Reference/Global_Objects/Array/filter 주어진 함수의 조건을 통과하는 배열의 모든 요소들을 모아 새로운 배열을 반환 배열의 각 요소들에 대해 한번 callback함수가 주어지고, 콜백함수가 true를 반환하는 각 요소들의 새로운 배열을 반환합니다. |
Array.prototype.map() - https://developer.mozilla.org/ko/docs/Web/JavaScript/Reference/Global_Objects/Array/map 배열 내의 모든 요소 각각에 대하여 주어진 함수를 호출한 결과를 모아 새로운 배열을 반환 map은 콜백함수를 각각의 요소에 대해 한번씩 순서대로 불러 그 함수의 반환값으로 새로운 배열을 만든다. |
Array.prototype.sort() - https://developer.mozilla.org/ko/docs/Web/JavaScript/Reference/Global_Objects/Array/sort 배열의 요소를 적절한 위치에 정렬한 후 그 배열을 반환 매개변수로 compareFunction이 제공되지 않으면 요소를 문자열로 변환하고 유니코드 순으로 문자열을 정렬합니다. compareFunction이 제공되면 배열 요소들은 이 함수의 반환값에 따라서 정렬됩니다. a, b가 비교되는 요소라면, compareFunction(a, b)에서 0보다 작은 값 반환 → a가 먼저 나옴 0보다 큰 값 반환 → b가 먼저 나옴 |
Array.prototype.reduce() - https://developer.mozilla.org/ko/docs/Web/JavaScript/Reference/Global_Objects/Array/Reduce 배열의 각 요소에 대해 주어진 리듀서(reducer) 함수를 실행하고, 하나의 결과값을 반환 reduce() 함수는 크게 두 가지 매개변수를 받습니다 : callback함수와 initialValue입니다. Callback함수에서도 네 가지 인수를 받습니다 accumulator : 누산기는 콜백의 반환값을 누적합니다. currentValue : 처리할 현재 요소 currentIndex : 처리할 현재 요소의 인덱스 arrya : reduce()를 호출한 배열 initialValue는 callback의 최초 호출에서 첫 번째 인수에 주는 값입니다. 초기값을 제공하지 않으면 배열의 첫 번째 요소를 사용합니다. |
console.table() - 테이블 형태로 표시한다. |
console과 관련된 메서드와 콜백함수에 대한 개념을 확실히 해야할 것 같네요
아무튼 이렇게 배열과 관련된 메서드 filter, map, sort, reduce를 공부할 수 있었습니다.
오늘은 여기까지 입니다.
읽어주셔서 감사하고 좋은 하루 보내시길 바랍니다.
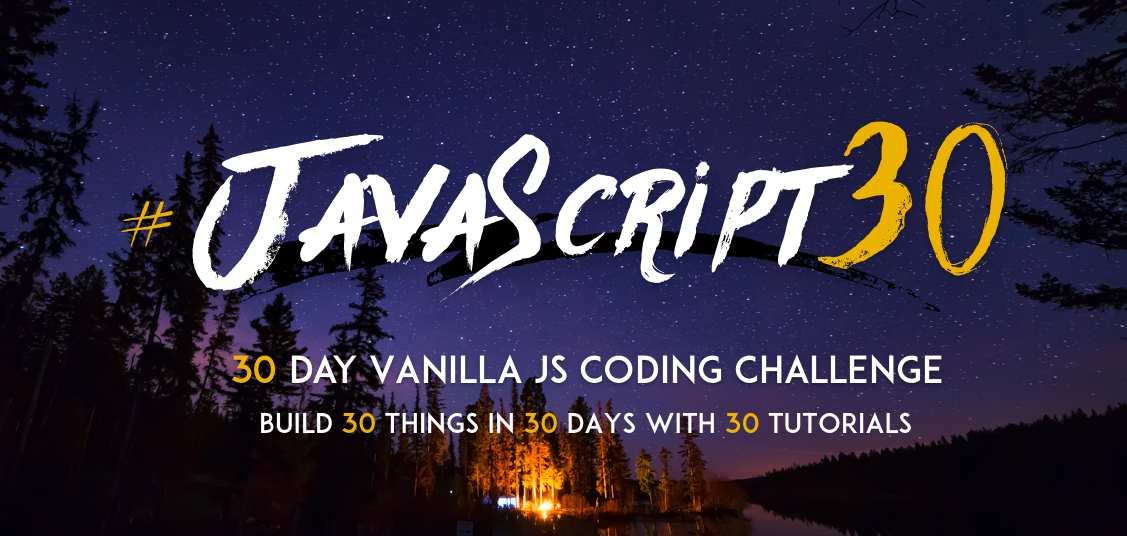
안녕하세요 오늘도 자스 연습을 해보도록 하겠습니다
JavaScript 30
Build 30 things with vanilla JS in 30 days with 30 tutorials
javascript30.com
목차 바로가기
1. 오늘의 과제는? |
2. 내가 만든 파일 |
3. finished파일 리뷰 |
4. 배운 점 & 느낀 점 |
1. 오늘의 과제는?
오늘은 몇가지 배열 같은 데이터들을 이용해서 자바스크립트 배열과 관련된 함수를 써보는 과제입니다.
start 파일의 주석을 읽어보시면 데이터를 가지고 뭘 해야하는지, 어떤 함수를 써야하는지까지 나와있습니다. 이를 보시고 finished 파일처럼 작동하게 만드시면 됩니다.
start.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Array Cardio 💪</title>
</head>
<body>
<p><em>Psst: have a look at the JavaScript Console</em> 💁</p>
<script>
// Get your shorts on - this is an array workout!
// ## Array Cardio Day 1
// Some data we can work with
const inventors = [
{ first: 'Albert', last: 'Einstein', year: 1879, passed: 1955 },
{ first: 'Isaac', last: 'Newton', year: 1643, passed: 1727 },
{ first: 'Galileo', last: 'Galilei', year: 1564, passed: 1642 },
{ first: 'Marie', last: 'Curie', year: 1867, passed: 1934 },
{ first: 'Johannes', last: 'Kepler', year: 1571, passed: 1630 },
{ first: 'Nicolaus', last: 'Copernicus', year: 1473, passed: 1543 },
{ first: 'Max', last: 'Planck', year: 1858, passed: 1947 },
{ first: 'Katherine', last: 'Blodgett', year: 1898, passed: 1979 },
{ first: 'Ada', last: 'Lovelace', year: 1815, passed: 1852 },
{ first: 'Sarah E.', last: 'Goode', year: 1855, passed: 1905 },
{ first: 'Lise', last: 'Meitner', year: 1878, passed: 1968 },
{ first: 'Hanna', last: 'Hammarström', year: 1829, passed: 1909 }
];
const people = ['Beck, Glenn', 'Becker, Carl', 'Beckett, Samuel', 'Beddoes, Mick', 'Beecher, Henry', 'Beethoven, Ludwig', 'Begin, Menachem', 'Belloc, Hilaire', 'Bellow, Saul', 'Benchley, Robert', 'Benenson, Peter', 'Ben-Gurion, David', 'Benjamin, Walter', 'Benn, Tony', 'Bennington, Chester', 'Benson, Leana', 'Bent, Silas', 'Bentsen, Lloyd', 'Berger, Ric', 'Bergman, Ingmar', 'Berio, Luciano', 'Berle, Milton', 'Berlin, Irving', 'Berne, Eric', 'Bernhard, Sandra', 'Berra, Yogi', 'Berry, Halle', 'Berry, Wendell', 'Bethea, Erin', 'Bevan, Aneurin', 'Bevel, Ken', 'Biden, Joseph', 'Bierce, Ambrose', 'Biko, Steve', 'Billings, Josh', 'Biondo, Frank', 'Birrell, Augustine', 'Black, Elk', 'Blair, Robert', 'Blair, Tony', 'Blake, William'];
// Array.prototype.filter()
// 1. Filter the list of inventors for those who were born in the 1500's
// Array.prototype.map()
// 2. Give us an array of the inventors' first and last names
// Array.prototype.sort()
// 3. Sort the inventors by birthdate, oldest to youngest
// Array.prototype.reduce()
// 4. How many years did all the inventors live?
// 5. Sort the inventors by years lived
// 6. create a list of Boulevards in Paris that contain 'de' anywhere in the name
// https://en.wikipedia.org/wiki/Category:Boulevards_in_Paris
// 7. sort Exercise
// Sort the people alphabetically by last name
// 8. Reduce Exercise
// Sum up the instances of each of these
const data = ['car', 'car', 'truck', 'truck', 'bike', 'walk', 'car', 'van', 'bike', 'walk', 'car', 'van', 'car', 'truck' ];
</script>
</body>
</html>
보시는바와 같이 오늘은 html과 css가 중요하지 않습니다. Only JS에만 집중하시면 될거같습니다.
2. 내가 만든 파일
// Array.prototype.filter()
// 1. Filter the list of inventors for those who were born in the 1500's
const inventors_1500 = inventors.filter(inventor => {
if(inventor.year >= 1500 && inventor.year < 1600) return inventor;
});
console.log(inventors_1500);
우선 첫번째로 1500년대에 태어난 발명가들의 리스트를 필터링하는 것입니다. 화살표함수를 통해서 1500~1599년에 태어난 사람을 선별해서 출력했습니다. 필터 메서드를 통해서 리스트를 반환하는 것을 확인할 수 있었습니다.
// Array.prototype.map()
// 2. Give us an array of the inventors' first and last names
const inventorsName = inventors.map(inventor => {
return [inventor.first, inventor.last];
});
console.log(inventorsName);
발명가의 첫번째 마지막 이름의 배열을 출력하는 것입니다. map() 메서드를 통해서 배열의 모든 요소에 접근할 수 있었고, 각 요소의 first와 last를 가져와서 배열로 반환했습니다. 나중에 완성본 파일 보니까 그냥 중첩배열말고 문자열의 배열로 해도 괜찮았나 봅니다.
// Array.prototype.sort()
// 3. Sort the inventors by birthdate, oldest to youngest
const sortedInventors = inventors.sort(function (a, b) {
if(a.year > b.year) {
return -1;
}
else if(a.year < b.year) {
return 1;
}
else {
return 0;
}
});
console.log(sortedInventors);
태어난 년도를 활용해서 오름차순이나 내림차순으로 정렬하면 됩니다. 저는 내림차순으로 정렬하기 위해서 a.year가 더 클 때 -1 을 반환해서 a가 먼저 오게 만들었습니다.
// Array.prototype.reduce()
// 4. How many years did all the inventors live?
const inventorsLivedYears = inventors.reduce(function (total, inventor) {
return total + (inventor.passed - inventor.year);
}, 0);
console.log(inventorsLivedYears);
다음으로 reduce() 메서드입니다. 발명가들이 살았던 기간들의 총합을 구하면 됩니다.
reduce는 좀 이해가 안갔었는데 우리가 1부터 10까지의 합을 구할 때 변수 sum같은 것을 이용해서 값들을 쌓는식으로 총합을 구하는데 그런것과 비슷한 느낌인거같습니다.
그래서 total에 inventor가 살았던 기간(inventor.passed - inventor.year)를 모두 더합니다. 그리고 total의 초기값으로 0으로 준것을 확인할 수 있습니다.
// 5. Sort the inventors by years lived
const sortedByLivedYears = inventors.sort(function (a, b) {
aLived = a.passed - a.year;
bLived = b.passed - b.year;
if(aLived > bLived) {
return -1;
}
else if(aLived < bLived) {
return 1;
}
else {
return 0;
}
});
console.log(sortedByLivedYears);
살았던 기간을 기준으로 sort를 하면 됩니다. 저는 내림차순으로 했습니다.
6번째는 어떻게 하는지 몰라서 못했습니다.
// 7. sort Exercise
// Sort the people alphabetically by last name
const sortedPeople = people.sort((previOne, nextOne) => {
const a = previOne.split(", ");
const b = nextOne.split(", ");
if(a[0] > b[0]) {
return 1;
}
else if(a[0] < b[0]) {
return -1;
}
else {
return 0;
}
});
console.log(sortedPeople);
Last name을 기준으로 알파벳순으로 정렬하면 됩니다. 우선 people배열이
const people = ['Beck, Glenn', 'Becker, Carl', 'Beckett, Samuel', 'Beddoes, Mick', 'Beecher, Henry', 'Beethoven, Ludwig', 'Begin, Menachem', 'Belloc, Hilaire', 'Bellow, Saul', 'Benchley, Robert', 'Benenson, Peter', 'Ben-Gurion, David', 'Benjamin, Walter', 'Benn, Tony', 'Bennington, Chester', 'Benson, Leana', 'Bent, Silas', 'Bentsen, Lloyd', 'Berger, Ric', 'Bergman, Ingmar', 'Berio, Luciano', 'Berle, Milton', 'Berlin, Irving', 'Berne, Eric', 'Bernhard, Sandra', 'Berra, Yogi', 'Berry, Halle', 'Berry, Wendell', 'Bethea, Erin', 'Bevan, Aneurin', 'Bevel, Ken', 'Biden, Joseph', 'Bierce, Ambrose', 'Biko, Steve', 'Billings, Josh', 'Biondo, Frank', 'Birrell, Augustine', 'Black, Elk', 'Blair, Robert', 'Blair, Tony', 'Blake, William'];
이렇게 생겼습니다. 따라서 저기 보이는 맨 처음 이름인 "Beck, Glenn"을 예를 들자면 split(", ")을 통해서 Beck과 Glenn으로 나눠서 Beck을 선택해서 이것을 기준으로 정렬해야합니다.
따라서 split(", ")을 하게되면 ["Beck", "Glenn"] 이라는 배열이 반환될 것이고 따라서 배열의 인덱스 0번째를 선택해야합니다.
제가 쓴 코드에서도 잘 반영되어 있습니다. a와 b에서 각각 두개의 단어배열을 받아서 0번째 요소로 알파벳순서를 비교합니다. 그렇게 해서 사전상으로 작은게 먼저 오도록 정렬했습니다.
// 8. Reduce Exercise
// Sum up the instances of each of these
const data = ['car', 'car', 'truck', 'truck', 'bike', 'walk', 'car', 'van', 'bike', 'walk', 'car', 'van', 'car', 'truck' ];
const sumUpData = data.reduce(function(obj, item) {
if (!obj[item]) {
obj[item] = 0;
}
obj[item]++;
return obj;
}, {});
console.log(sumUpData);
data 배열을 요약하는 과제인데 어떻게 하는지 몰라서 finished 버전을 보고 이해하였습니다. 3번째 단락 finished파일 리뷰에서 설명을 해보겠습니다.
3. finished파일 리뷰
// Array.prototype.filter()
// 1. Filter the list of inventors for those who were born in the 1500's
const fifteen = inventors.filter(inventor => (inventor.year >= 1500 && inventor.year < 1600));
console.table(fifteen);
화살표 함수를 사용해서 더 간단하게 결과를 출력한 것을 볼 수 있습니다.
// Array.prototype.map()
// 2. Give us an array of the inventor first and last names
const fullNames = inventors.map(inventor => `${inventor.first} ${inventor.last}`);
console.log(fullNames);
map을 통해서 배열의 요소들을 하나하나 돌면서 리터럴 문자열을 이용해서 간단하게 결과를 출력했습니다.
// Array.prototype.sort()
// 3. Sort the inventors by birthdate, oldest to youngest
// const ordered = inventors.sort(function(a, b) {
// if(a.year > b.year) {
// return 1;
// } else {
// return -1;
// }
// });
const ordered1 = inventors.sort((a, b) => a.year > b.year ? 1 : -1);
console.table(ordered1);
삼항연산자를 통해서 더 a.year가 더 크면 1을 반환해서 b가 먼저 나올 수 있게 sort() 메서드를 쓴 것을 볼 수 있습니다. 그리고 console.table() 를 쓴 것을 볼 수 있습니다.
// Array.prototype.reduce()
// 4. How many years did all the inventors live?
const totalYears = inventors.reduce((total, inventor) => {
return total + (inventor.passed - inventor.year);
}, 0);
console.log(totalYears);
설명 생략
// 5. Sort the inventors by years lived
const oldest = inventors.sort(function(a, b) {
const lastInventor = a.passed - a.year;
const nextInventor = b.passed - b.year;
return lastInventor > nextInventor ? -1 : 1;
});
console.table(oldest);
제가 한 것에서 삼항 연산자를 통해서 결과를 얻어낸 모습입니다.
삼항연산자가 확실히 더 깔끔해 보이는 것을 볼 수 있습니다. 저도 삼항연산자를 자주 써봐야겠네요
// 6. create a list of Boulevards in Paris that contain 'de' anywhere in the name
// https://en.wikipedia.org/wiki/Category:Boulevards_in_Paris
// const category = document.querySelector('.mw-category');
// const links = Array.from(category.querySelectorAll('a'));
// const de = links
// .map(link => link.textContent)
// .filter(streetName => streetName.includes('de'));
저 사이트에 들어가서 주석처리된 코드를 입력하시면 이름에 'de' 가 들어간 Boulevards in Paris의 배열을 얻을 수 있습니다.
.mv-category 클래스를 써서 각 a태그들이 담겨있는 박스를 가져와서 category상수에 저장합니다. 그리고 그 category에서 a태그를 가진 객체들을 모두 가져와서 배열로 만든 것을 links에 저장합니다.
links에서 map을 통해서 모든 배열요소들을 돌면서 a태그 객체에 담겨있는 textContent들의 배열을 만듭니다.
그리고 filter 메서드를 통해서 각 요소들에서 'de'가 들어간 요소들의 배열을 반환합니다.
직접 해당 사이트에서 크롬개발도구(F12)를 써서 해보시면 이해가 더 쉬울것입니다.
// 7. sort Exercise
// Sort the people alphabetically by last name
const alpha = people.sort((lastOne, nextOne) => {
const [aLast, aFirst] = lastOne.split(', ');
const [bLast, bFirst] = nextOne.split(', ');
return aLast > bLast ? 1 : -1;
});
console.log(alpha);
설명 생략
// 8. Reduce Exercise
// Sum up the instances of each of these
const data = ['car', 'car', 'truck', 'truck', 'bike', 'walk', 'car', 'van', 'bike', 'walk', 'car', 'van', 'car', 'truck' ];
const sumUpData = data.reduce(function(obj, item) {
if (!obj[item]) {
obj[item] = 0;
}
obj[item]++;
return obj;
}, {});
console.log(sumUpData);
data 배열을 요약하는 객체를 출력하면 됩니다.
우선 function(obj, item) 으로 진행했는데 여기서 obj의 초기값으로 {}를 줬습니다. 따라서 객체가 될 수 있었습니다.
그리고 item에는 각 배열의 요소들이 담기게 될 것인데 예를 들어 맨 처음엔 obj['car']가 될 수 있습니다.
하지만 객체에 없었던 것이므로 if 조건에 맞아떨어집니다. 따라서 obj[item] = 0; 을 통해서 객체의 요소로 설정되고 obj[item]++; 로 개수가 설정됩니다.
그리고 reduce() 메서드 이므로 계속 중첩되고 결과적으로 요약이 출력됩니다.
4. 배운 점 & 느낀 점
em태그 - 강조된 텍스트를 표현할 때 사용 - http://tcpschool.com/html-tags/em |
Array.prototype.filter() -https://developer.mozilla.org/ko/docs/Web/JavaScript/Reference/Global_Objects/Array/filter 주어진 함수의 조건을 통과하는 배열의 모든 요소들을 모아 새로운 배열을 반환 배열의 각 요소들에 대해 한번 callback함수가 주어지고, 콜백함수가 true를 반환하는 각 요소들의 새로운 배열을 반환합니다. |
Array.prototype.map() - https://developer.mozilla.org/ko/docs/Web/JavaScript/Reference/Global_Objects/Array/map 배열 내의 모든 요소 각각에 대하여 주어진 함수를 호출한 결과를 모아 새로운 배열을 반환 map은 콜백함수를 각각의 요소에 대해 한번씩 순서대로 불러 그 함수의 반환값으로 새로운 배열을 만든다. |
Array.prototype.sort() - https://developer.mozilla.org/ko/docs/Web/JavaScript/Reference/Global_Objects/Array/sort 배열의 요소를 적절한 위치에 정렬한 후 그 배열을 반환 매개변수로 compareFunction이 제공되지 않으면 요소를 문자열로 변환하고 유니코드 순으로 문자열을 정렬합니다. compareFunction이 제공되면 배열 요소들은 이 함수의 반환값에 따라서 정렬됩니다. a, b가 비교되는 요소라면, compareFunction(a, b)에서 0보다 작은 값 반환 → a가 먼저 나옴 0보다 큰 값 반환 → b가 먼저 나옴 |
Array.prototype.reduce() - https://developer.mozilla.org/ko/docs/Web/JavaScript/Reference/Global_Objects/Array/Reduce 배열의 각 요소에 대해 주어진 리듀서(reducer) 함수를 실행하고, 하나의 결과값을 반환 reduce() 함수는 크게 두 가지 매개변수를 받습니다 : callback함수와 initialValue입니다. Callback함수에서도 네 가지 인수를 받습니다 accumulator : 누산기는 콜백의 반환값을 누적합니다. currentValue : 처리할 현재 요소 currentIndex : 처리할 현재 요소의 인덱스 arrya : reduce()를 호출한 배열 initialValue는 callback의 최초 호출에서 첫 번째 인수에 주는 값입니다. 초기값을 제공하지 않으면 배열의 첫 번째 요소를 사용합니다. |
console.table() - 테이블 형태로 표시한다. |
console과 관련된 메서드와 콜백함수에 대한 개념을 확실히 해야할 것 같네요
아무튼 이렇게 배열과 관련된 메서드 filter, map, sort, reduce를 공부할 수 있었습니다.
오늘은 여기까지 입니다.
읽어주셔서 감사하고 좋은 하루 보내시길 바랍니다.