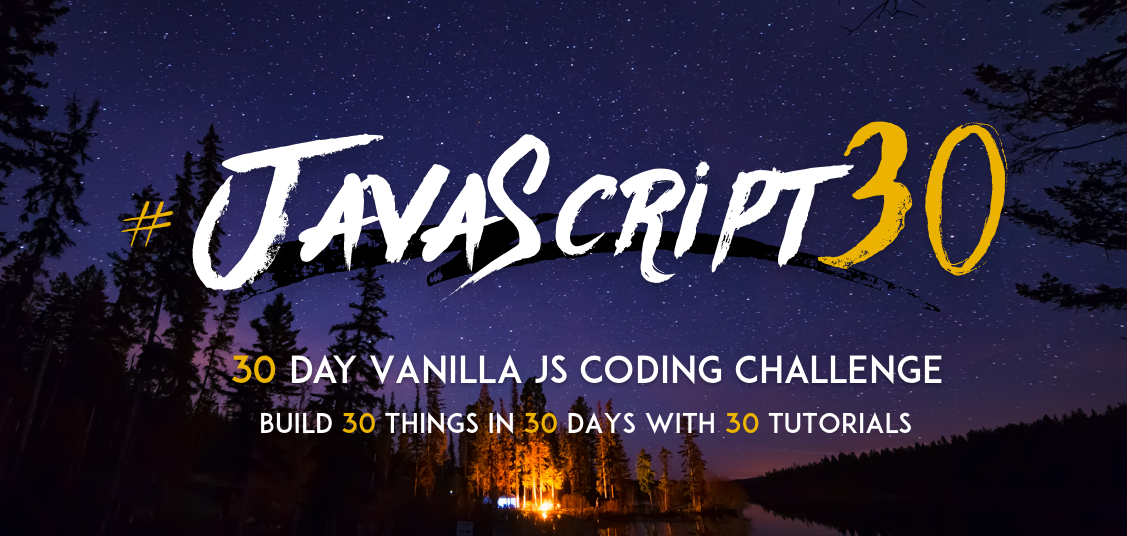
안녕하세요 오늘도 자스 연습을 해보도록 하겠습니다
1. 오늘의 과제는?
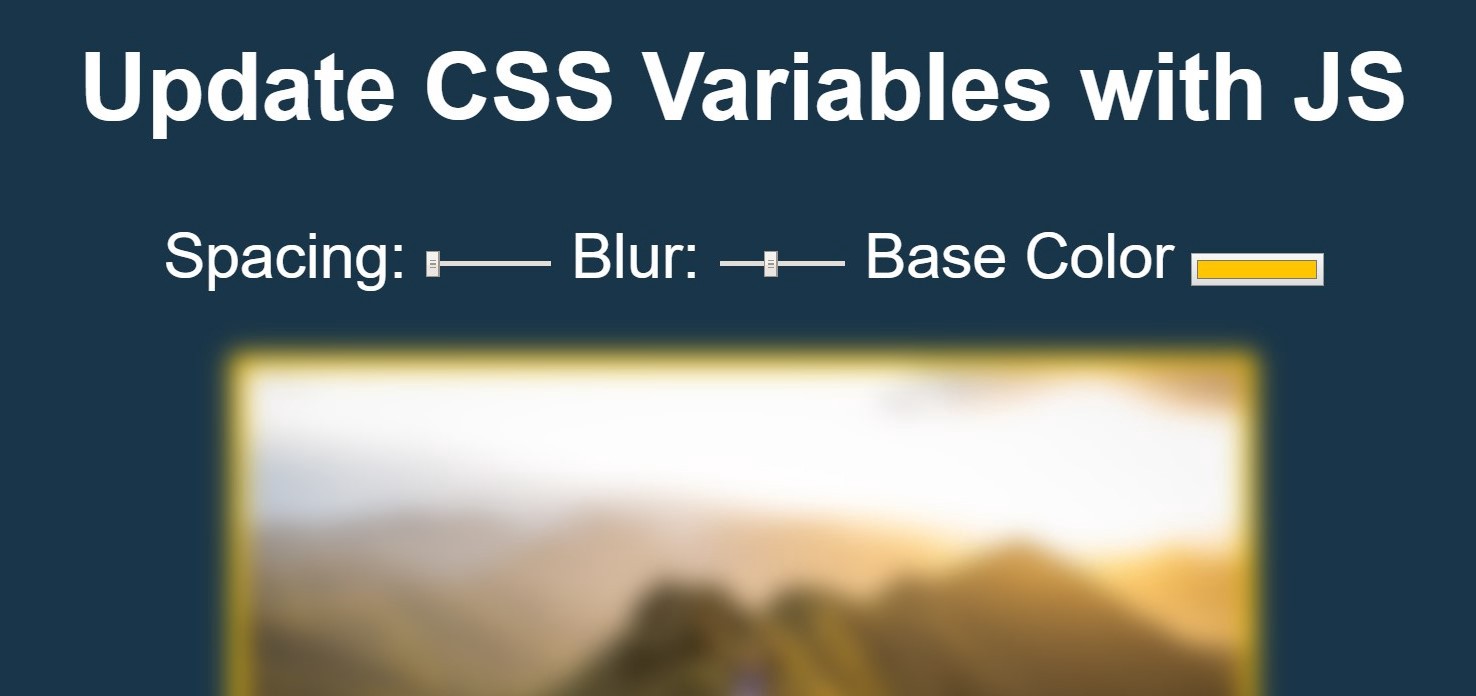
오늘은 js를 사용해서 실시간으로 CSS변화를 만드는 애플리케이션을 만들어야 합니다.
우선 start.html 파일은 이러합니다.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Scoped CSS Variables and JS</title>
</head>
<body>
<h2>Update CSS Variables with <span class='hl'>JS</span></h2>
<div class="controls">
<label for="spacing">Spacing:</label>
<input id="spacing" type="range" name="spacing" min="10" max="200" value="10" data-sizing="px">
<label for="blur">Blur:</label>
<input id="blur" type="range" name="blur" min="0" max="25" value="10" data-sizing="px">
<label for="base">Base Color</label>
<input id="base" type="color" name="base" value="#ffc600">
</div>
<img src="https://source.unsplash.com/7bwQXzbF6KE/800x500">
<style>
/*
misc styles, nothing to do with CSS variables
*/
body {
text-align: center;
background: #193549;
color: white;
font-family: 'helvetica neue', sans-serif;
font-weight: 100;
font-size: 50px;
}
.controls {
margin-bottom: 50px;
}
input {
width: 100px;
}
</style>
<script>
</script>
</body>
</html>
여기서 시작해서 finished 버전과 같게 동작하게 만들면 됩니다.
2. 내가 만든 파일
CSS 파일
body {
text-align: center;
background-color: #193549;
color: white;
font-weight: 100;
font-size: 50px;
}
.controls {
margin-bottom: 50px;
}
input {
width: 100px;
}
img {
filter: blur(10px);
background: #ffc600;
padding: 10px;
}
.h1 {
color: #ffc600;
}
start.html 파일에 각 input 태그에 초기값(속성 value를 보면된다)들이 설정되어 있는데 이것들은 CSS에서 초기화해줘야 합니다. 따라서 img 태그의 css 초기화를 진행해주고 클래스h1의 span태그의 글자 색도 초기화를 해줬습니다.
JS파일
const spacing = document.querySelector('#spacing'); //패딩값
const blur = document.querySelector('#blur'); //블러
//base 색에 따라서 색깔변경
const base = document.querySelector('#base');
const h1 = document.querySelector('.h1');
const img = document.querySelector('img');
//패딩값
spacing.addEventListener('input', () => {
let spacingVal = spacing.value;
img.style.padding = `${spacingVal}px`;
});
//블러
blur.addEventListener('input', () => {
let blurVal = blur.value;
img.style.filter = `blur(${blurVal}px)`;
});
//색깔
base.addEventListener('input', () => {
let baseColor = base.value;
h1.style.color = baseColor;
img.style.background = baseColor;
});
먼저 각 변화가 있는 객체들을 모두 가져왔고 각자 값이 변화할 때 마다 input 이벤트가 일어납니다. 그래서 addEventListener를 이용해서 input 이벤트가 일어날 때마다 img와 .h1의 style변화를 일으킵니다.
3. finished파일 리뷰
CSS파일
:root {
--base: #ffc600;
--spacing: 10px;
--blur: 10px;
}
우선 통일성을 위해 :root 변수를 사용한 것을 볼 수 있다. 이를 활용해 유지보수를 편하게 할 수 있다.
img {
padding: var(--spacing);
background: var(--base);
filter: blur(var(--blur));
}
.hl {
color: var(--base);
}
:root 변수를 써서 각 태그들에 초기값을 지정한 모습이다.
JS파일
const inputs = document.querySelectorAll('.controls input');
.controls에 input인 태그들 모두를 가져온 코드이다. 배열형태로 저장될 것이다.
function handleUpdate() {
const suffix = this.dataset.sizing || '';
document.documentElement.style.setProperty(`--${this.name}`, this.value + suffix);
}
dataset을 사용하면 data-*의 속성들이 불러지는데 여기서 data-sizing인 속성을 가져온 것이다. 위의 슬라이드 바에서 사용되었는데 "px"로 저장되어 있고 색깔을 지정하는 input태그에서는 data-sizing이 지정되어 있지 않다. 따라서 px로 지정된 슬라이더바 라면 suffix가 "px"로 저장될 것이고 그게 아니라면 공백이 저장될 것이다.
그리고 document.documentElement를 사용해서 root element를 호출했고 그것의 style.setProperty를 사용해서 속성을 지정했다.
inputs.forEach(input => input.addEventListener('change', handleUpdate));
inputs.forEach(input => input.addEventListener('mousemove', handleUpdate));
이벤트로 change와 mousemove를 사용했다
change를 사용하면 슬라이더바를 변화시키고 마우스를 멈췄을 때만 이벤트가 발생되어서 실시간으로 변화하는 값을 추적하기가 어렵다. 따라서 mousemove를 이용해서 실시간으로 변화하는 값을 얻어낼 수 있을 것이다.
4. 배운 점 & 느낀 점
html label 태그의 for 속성 - http://tcpschool.com/html-tags/label for 속성을 사용해 다른 요소와 결합할 수 있다. for 속성값이 결합하고자 하는 요소 id값과 같아야 한다. 이러한 label 태그의 텍스트를 클릭해도 label 과 연결된 요소를 바로 선택할 수 있어서 편하다. |
슬라이더바(input type range)의 실시간으로 바뀌는 값을 확인하려면 input 이벤트를 이용하면 된다. - https://developer.mozilla.org/ko/docs/Web/API/HTMLElement/input_event <input>, <select>, <textarea> 요소의 value 속성이 바뀔 때마다 발생한다. |
CSS로 블러효과 - https://webisfree.com/2019-07-09/css-filter-프로퍼티-사용하여-블러-효과-적용하기-blur CSS의 filter 프로퍼티를 사용해 포토샵의 일부 효과들을 웹에서도 적용할 수 있다. img { filter: blur(값); } - 값이 커질 수록 더 흐릿해져 보임 |
CSS :root 란 ? - https://designer-ej.tistory.com/entry/CSS-root-가상-클래스로-CSS-변수-다루기 CSS로 디자인을 할 때, 통일성을 위해 동일한 값을 자주 쓰게 되는데 이 때 유지보수를 쉽게 하기위해 :root 가상 클래스를 쓴다. :root를 사용해 최상위 요소에 변수를 선언하면 모든 요소에서 이 변수를 사용할 수 있다. 따라서 한번에 수정하기 용이하다. 변수 선언은 —이름: 속성 값 이런식으로 하는데 예를 들어 :root { —color-black: #3f454d; } 변수 사용은 var() 로 소괄호안에 속성 값을 입력한다. 예를 들어 .item { background-color: var(—color-black); } |
Document.documentElement - document의 root element를 반환 - https://developer.mozilla.org/en-US/docs/Web/API/Document/documentElement |
style.setProperty(propertyName, value, priority); - https://developer.mozilla.org/en-US/docs/Web/API/CSSStyleDeclaration/setProperty CSS 프로퍼티 설정을 할 수 있다. value와 priority는 옵션 값이다. |
여기까지 입니다
읽어주셔서 감사하고 좋은하루 보내시길 바랍니다.
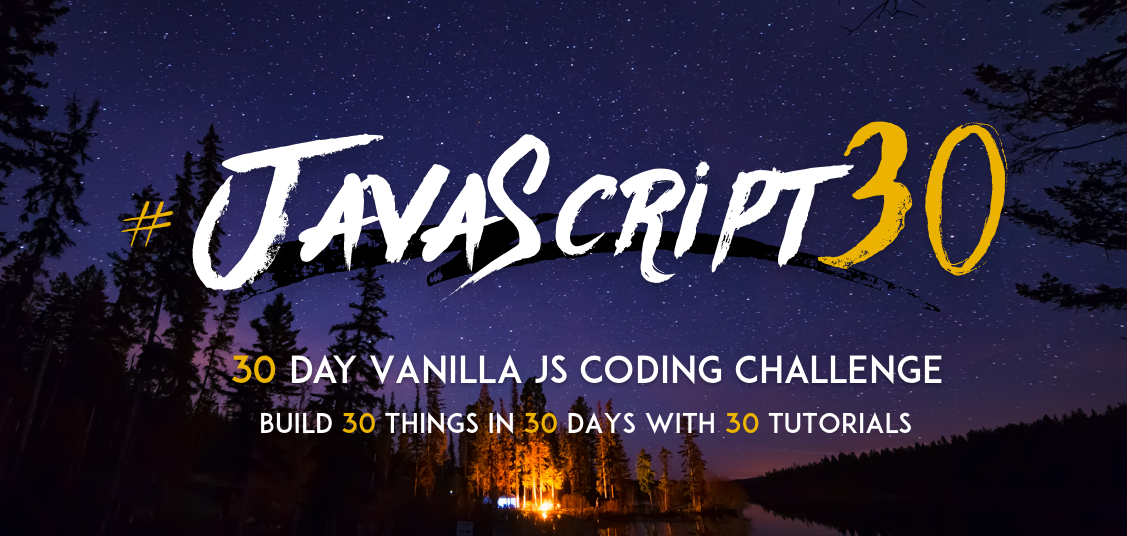
안녕하세요 오늘도 자스 연습을 해보도록 하겠습니다
1. 오늘의 과제는?
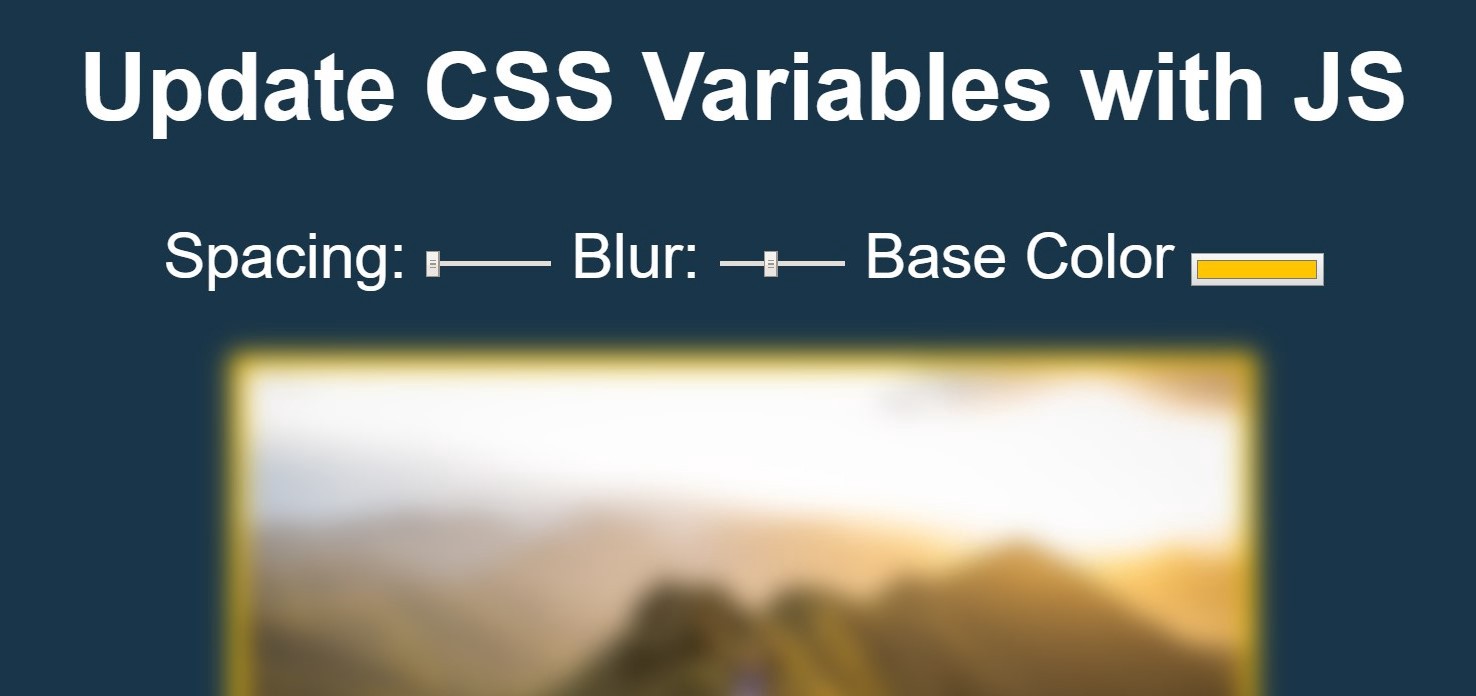
오늘은 js를 사용해서 실시간으로 CSS변화를 만드는 애플리케이션을 만들어야 합니다.
우선 start.html 파일은 이러합니다.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Scoped CSS Variables and JS</title>
</head>
<body>
<h2>Update CSS Variables with <span class='hl'>JS</span></h2>
<div class="controls">
<label for="spacing">Spacing:</label>
<input id="spacing" type="range" name="spacing" min="10" max="200" value="10" data-sizing="px">
<label for="blur">Blur:</label>
<input id="blur" type="range" name="blur" min="0" max="25" value="10" data-sizing="px">
<label for="base">Base Color</label>
<input id="base" type="color" name="base" value="#ffc600">
</div>
<img src="https://source.unsplash.com/7bwQXzbF6KE/800x500">
<style>
/*
misc styles, nothing to do with CSS variables
*/
body {
text-align: center;
background: #193549;
color: white;
font-family: 'helvetica neue', sans-serif;
font-weight: 100;
font-size: 50px;
}
.controls {
margin-bottom: 50px;
}
input {
width: 100px;
}
</style>
<script>
</script>
</body>
</html>
여기서 시작해서 finished 버전과 같게 동작하게 만들면 됩니다.
2. 내가 만든 파일
CSS 파일
body {
text-align: center;
background-color: #193549;
color: white;
font-weight: 100;
font-size: 50px;
}
.controls {
margin-bottom: 50px;
}
input {
width: 100px;
}
img {
filter: blur(10px);
background: #ffc600;
padding: 10px;
}
.h1 {
color: #ffc600;
}
start.html 파일에 각 input 태그에 초기값(속성 value를 보면된다)들이 설정되어 있는데 이것들은 CSS에서 초기화해줘야 합니다. 따라서 img 태그의 css 초기화를 진행해주고 클래스h1의 span태그의 글자 색도 초기화를 해줬습니다.
JS파일
const spacing = document.querySelector('#spacing'); //패딩값
const blur = document.querySelector('#blur'); //블러
//base 색에 따라서 색깔변경
const base = document.querySelector('#base');
const h1 = document.querySelector('.h1');
const img = document.querySelector('img');
//패딩값
spacing.addEventListener('input', () => {
let spacingVal = spacing.value;
img.style.padding = `${spacingVal}px`;
});
//블러
blur.addEventListener('input', () => {
let blurVal = blur.value;
img.style.filter = `blur(${blurVal}px)`;
});
//색깔
base.addEventListener('input', () => {
let baseColor = base.value;
h1.style.color = baseColor;
img.style.background = baseColor;
});
먼저 각 변화가 있는 객체들을 모두 가져왔고 각자 값이 변화할 때 마다 input 이벤트가 일어납니다. 그래서 addEventListener를 이용해서 input 이벤트가 일어날 때마다 img와 .h1의 style변화를 일으킵니다.
3. finished파일 리뷰
CSS파일
:root {
--base: #ffc600;
--spacing: 10px;
--blur: 10px;
}
우선 통일성을 위해 :root 변수를 사용한 것을 볼 수 있다. 이를 활용해 유지보수를 편하게 할 수 있다.
img {
padding: var(--spacing);
background: var(--base);
filter: blur(var(--blur));
}
.hl {
color: var(--base);
}
:root 변수를 써서 각 태그들에 초기값을 지정한 모습이다.
JS파일
const inputs = document.querySelectorAll('.controls input');
.controls에 input인 태그들 모두를 가져온 코드이다. 배열형태로 저장될 것이다.
function handleUpdate() {
const suffix = this.dataset.sizing || '';
document.documentElement.style.setProperty(`--${this.name}`, this.value + suffix);
}
dataset을 사용하면 data-*의 속성들이 불러지는데 여기서 data-sizing인 속성을 가져온 것이다. 위의 슬라이드 바에서 사용되었는데 "px"로 저장되어 있고 색깔을 지정하는 input태그에서는 data-sizing이 지정되어 있지 않다. 따라서 px로 지정된 슬라이더바 라면 suffix가 "px"로 저장될 것이고 그게 아니라면 공백이 저장될 것이다.
그리고 document.documentElement를 사용해서 root element를 호출했고 그것의 style.setProperty를 사용해서 속성을 지정했다.
inputs.forEach(input => input.addEventListener('change', handleUpdate));
inputs.forEach(input => input.addEventListener('mousemove', handleUpdate));
이벤트로 change와 mousemove를 사용했다
change를 사용하면 슬라이더바를 변화시키고 마우스를 멈췄을 때만 이벤트가 발생되어서 실시간으로 변화하는 값을 추적하기가 어렵다. 따라서 mousemove를 이용해서 실시간으로 변화하는 값을 얻어낼 수 있을 것이다.
4. 배운 점 & 느낀 점
html label 태그의 for 속성 - http://tcpschool.com/html-tags/label for 속성을 사용해 다른 요소와 결합할 수 있다. for 속성값이 결합하고자 하는 요소 id값과 같아야 한다. 이러한 label 태그의 텍스트를 클릭해도 label 과 연결된 요소를 바로 선택할 수 있어서 편하다. |
슬라이더바(input type range)의 실시간으로 바뀌는 값을 확인하려면 input 이벤트를 이용하면 된다. - https://developer.mozilla.org/ko/docs/Web/API/HTMLElement/input_event <input>, <select>, <textarea> 요소의 value 속성이 바뀔 때마다 발생한다. |
CSS로 블러효과 - https://webisfree.com/2019-07-09/css-filter-프로퍼티-사용하여-블러-효과-적용하기-blur CSS의 filter 프로퍼티를 사용해 포토샵의 일부 효과들을 웹에서도 적용할 수 있다. img { filter: blur(값); } - 값이 커질 수록 더 흐릿해져 보임 |
CSS :root 란 ? - https://designer-ej.tistory.com/entry/CSS-root-가상-클래스로-CSS-변수-다루기 CSS로 디자인을 할 때, 통일성을 위해 동일한 값을 자주 쓰게 되는데 이 때 유지보수를 쉽게 하기위해 :root 가상 클래스를 쓴다. :root를 사용해 최상위 요소에 변수를 선언하면 모든 요소에서 이 변수를 사용할 수 있다. 따라서 한번에 수정하기 용이하다. 변수 선언은 —이름: 속성 값 이런식으로 하는데 예를 들어 :root { —color-black: #3f454d; } 변수 사용은 var() 로 소괄호안에 속성 값을 입력한다. 예를 들어 .item { background-color: var(—color-black); } |
Document.documentElement - document의 root element를 반환 - https://developer.mozilla.org/en-US/docs/Web/API/Document/documentElement |
style.setProperty(propertyName, value, priority); - https://developer.mozilla.org/en-US/docs/Web/API/CSSStyleDeclaration/setProperty CSS 프로퍼티 설정을 할 수 있다. value와 priority는 옵션 값이다. |
여기까지 입니다
읽어주셔서 감사하고 좋은하루 보내시길 바랍니다.